How to Optimize Your Android App for Performance and Speed
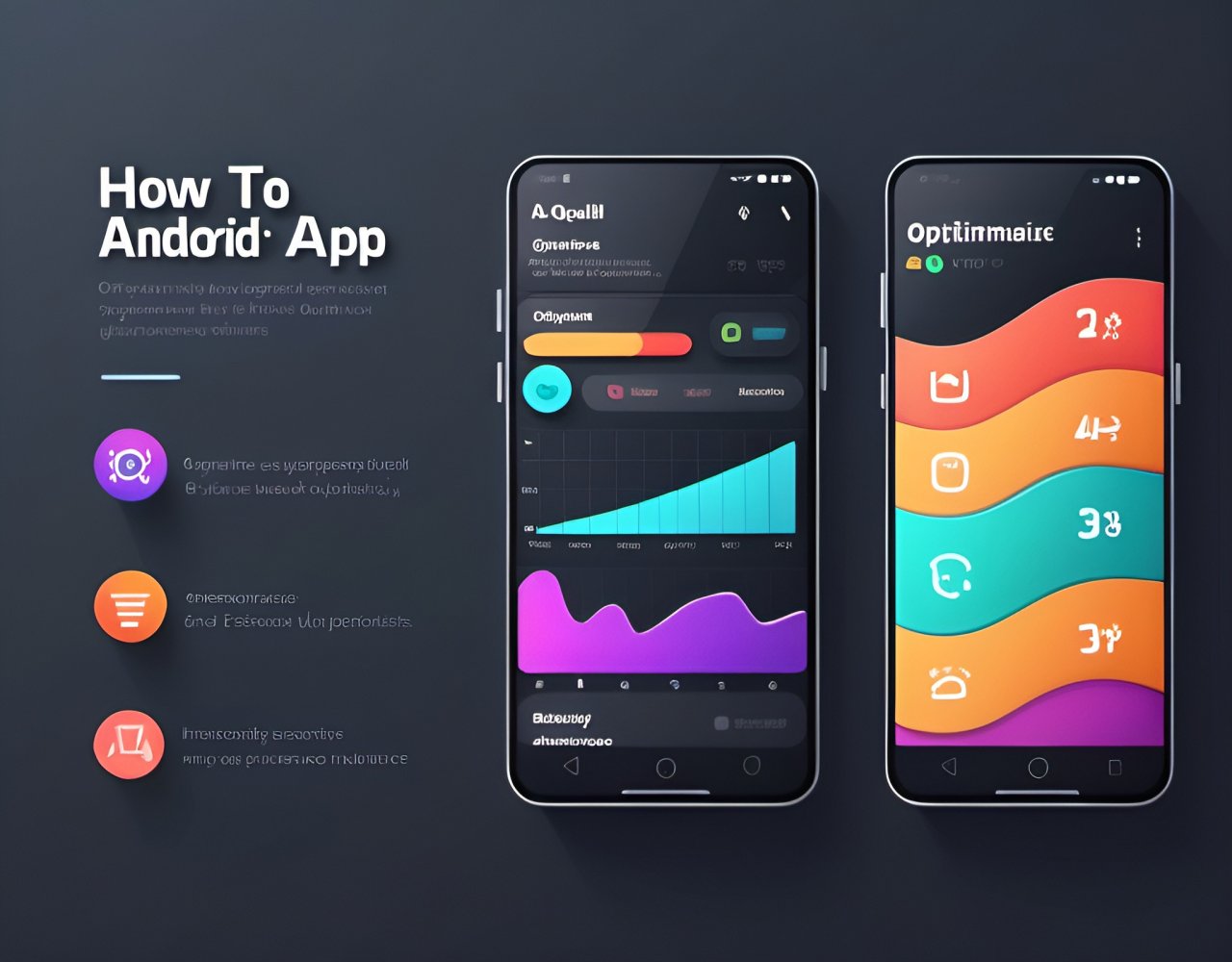
In today’s fast-paced world, users expect apps to be quick, responsive, and efficient. Slow load times, laggy performance, or crashes can drive users away, leading to poor app ratings and decreased engagement. Optimizing your Android app for performance and speed isn’t just a nice-to-have — it's essential for retaining users and staying competitive.
Here’s a step-by-step guide to optimizing your Android app for maximum performance and speed.
1. Use Efficient Data Structures
Choosing the right data structures is fundamental to improving app performance. Efficient data structures, such as ArrayList
, SparseArray
, or HashMap
, can dramatically reduce memory consumption and processing time. For instance, SparseArray is more memory-efficient than a traditional HashMap
when dealing with primitive types like integers.
Tips:
- Avoid using heavyweight data structures unless necessary.
- Use SparseArray instead of
HashMap
for mapping integers to objects. - Favor
LinkedList
overArrayList
for dynamic data with frequent insertions and deletions.
2. Optimize Layout Hierarchies
Excessive or deeply nested layouts can slow down your app's rendering process. Every view added to the layout requires more time to process and display. Minimizing the depth of your layout hierarchy can result in faster rendering.
Tips:
- Use ConstraintLayout, which is more efficient and flexible than deeply nested LinearLayouts or RelativeLayouts.
- Leverage the Layout Inspector in Android Studio to analyze and optimize your view hierarchy.
- Use ViewStub for infrequently used views to delay loading until they are needed.
3. Minimize Overdraws
Overdraw occurs when a pixel is drawn multiple times before the final display. This can happen when views overlap unnecessarily. Minimizing overdraw can significantly improve the rendering speed of your UI.
Tips:
- Use Android Studio’s GPU Overdraw Debug tool to identify and fix overdraw issues.
- Flatten your UI hierarchy to reduce unnecessary view overlap.
- Set transparent backgrounds for views when possible to avoid multiple layers of drawing.
4. Optimize Bitmap Usage
Handling large bitmaps can lead to memory consumption spikes, causing your app to slow down or even crash due to OutOfMemoryErrors. Properly managing and optimizing bitmaps is crucial for performance.
Tips:
- Use BitmapFactory.Options to downsample images when loading them into memory, especially for high-resolution images.
- Load images asynchronously using libraries like Glide or Picasso to avoid blocking the main thread.
- Recycle bitmaps that are no longer in use to free up memory.
5. Reduce App Startup Time
Users expect apps to start quickly. A slow startup time can frustrate users and negatively affect retention. Optimizing your app’s startup time involves eliminating unnecessary processes and deferring operations that aren’t immediately required.
Tips:
- Use Android Profiler in Android Studio to identify bottlenecks during the startup phase.
- Defer or delay initialization of heavy components or services until they are needed.
- Avoid performing complex operations in the
onCreate
method of your main activity. - Use the Splash Screen API to improve the user experience while loading resources in the background.
6. Optimize Networking and Data Fetching
Inefficient network operations can slow down your app, especially when it relies on constant data fetching from servers. Optimizing how and when you fetch data can greatly improve both speed and responsiveness.
Tips:
- Use OkHttp or Retrofit for efficient network requests and handling.
- Implement caching to reduce unnecessary network calls. Store responses locally using tools like Room or the SQLite database.
- Batch network requests to avoid making multiple small requests in a short period.
- Use JobScheduler or WorkManager to schedule and manage background tasks intelligently.
7. Utilize Multithreading Efficiently
Offloading heavy tasks from the main thread can prevent your app from freezing or becoming unresponsive. Using proper multithreading techniques ensures that your app remains responsive, even during intensive processes.
Tips:
- Use AsyncTask (deprecated in newer Android versions) or, preferably, ExecutorService or RxJava for background tasks.
- Leverage Kotlin Coroutines for cleaner and more efficient asynchronous code.
- Offload heavy or long-running operations to background threads and only update the UI from the main thread.
8. Reduce APK Size
A large APK file can deter users from downloading your app and may also cause performance issues. Reducing the APK size ensures faster downloads and less storage usage on users’ devices.
Tips:
- Use Android App Bundles (AAB) to reduce APK size by delivering device-specific versions of your app.
- Enable ProGuard or R8 to remove unused code and shrink your APK.
- Compress large assets such as images and remove unused resources.
- Use vector drawables instead of multiple PNG files for scalable graphics.
9. Optimize Memory Usage
High memory usage can cause your app to slow down or even crash. It’s crucial to monitor and manage your app’s memory consumption to ensure a smooth user experience.
Tips:
- Use the Memory Profiler in Android Studio to detect memory leaks and over-allocated memory.
- Implement object pooling for frequently used objects to avoid creating new ones.
- Avoid holding references to activities or fragments in long-running tasks, as this can lead to memory leaks.
- Use WeakReferences when appropriate to prevent memory leaks.
10. Test and Profile Regularly
Finally, optimization is an ongoing process. Regularly testing your app using Android Studio’s built-in profiling tools can help you identify and fix performance bottlenecks. These tools allow you to monitor CPU usage, memory consumption, and network activity in real-time.
Tips:
- Use the CPU Profiler to monitor CPU-intensive operations and optimize code paths that consume too much processing power.
- The Network Profiler will help you track data usage and network request performance, ensuring that your app handles network traffic efficiently.
- Leverage LeakCanary to detect and fix memory leaks.
- Perform stress testing to see how your app performs under heavy usage conditions.
Conclusion
Optimizing your Android app for performance and speed is an essential aspect of delivering a great user experience. By following these strategies—efficient data structures, reducing layout complexity, optimizing memory usage, and more—you can create a fast, responsive, and reliable app. Regularly monitoring your app’s performance using Android Studio’s profiling tools ensures that you identify and resolve issues before they affect your users.
Investing time in performance optimization will not only enhance your app's quality but also contribute to better user retention, higher ratings, and ultimately, the success of your app.